This first picture is a top-down of the first puzzle in Area 4. It uses a new power-up where you use your yellow electricity which will be used to hit the yellow panels on the walls which will open up the barriers.
The second picture is the second puzzle where you must move blocks and use this power to solve this puzzle.
The third puzzle incorporates the mirrors from Area 2, pressure plates, lifts and barriers.
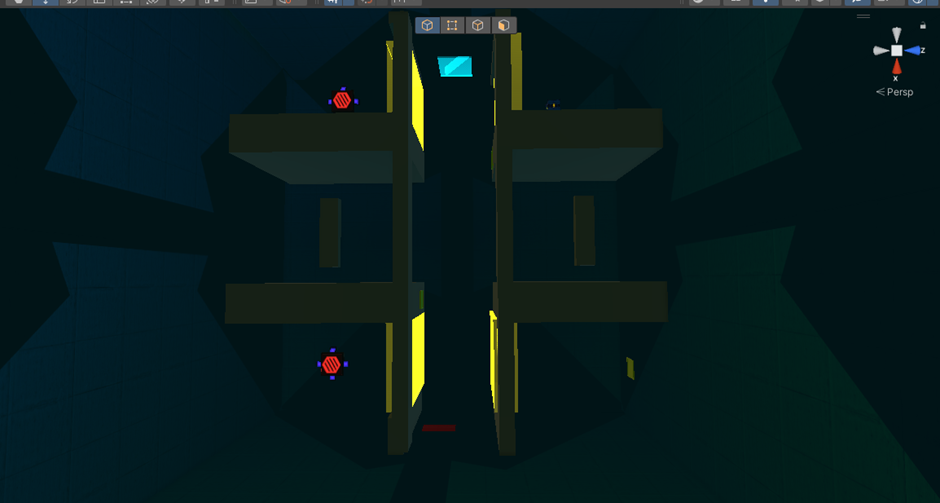
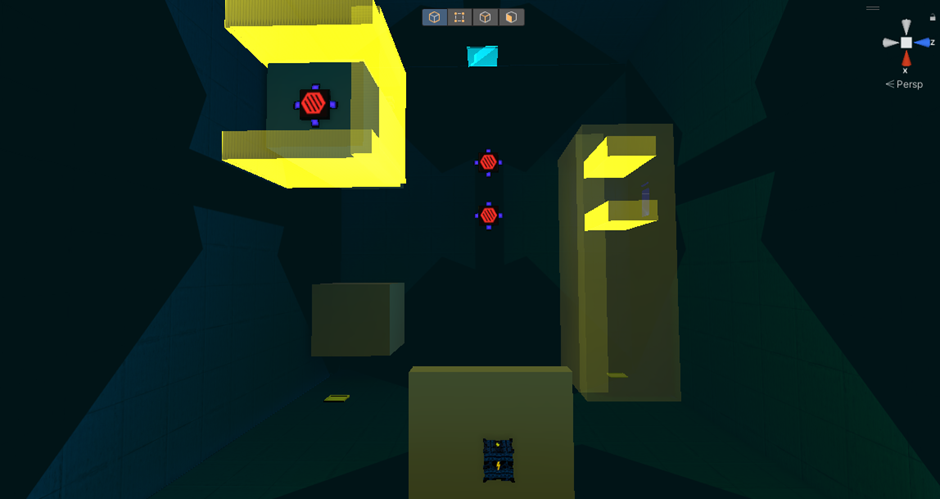
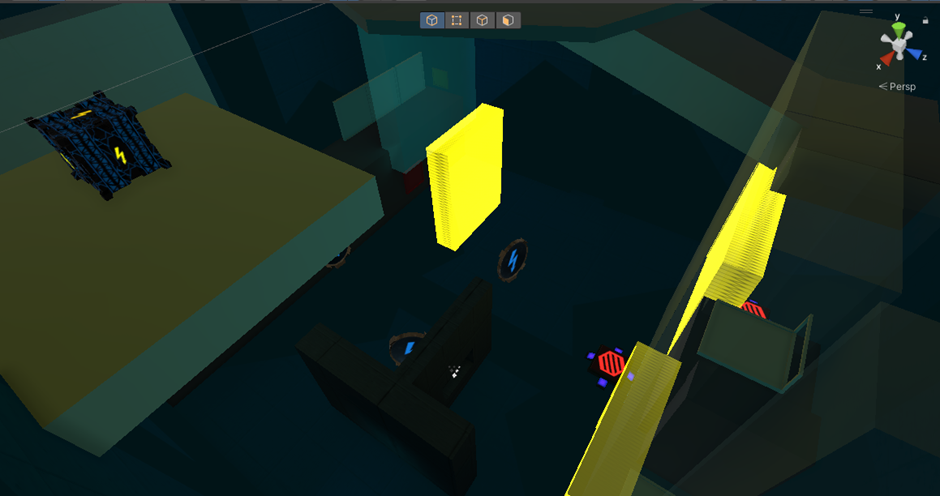
This is an overview of as section in Area 5’s first puzzle where you will use the magnet power to move the block from behind the glass wall.
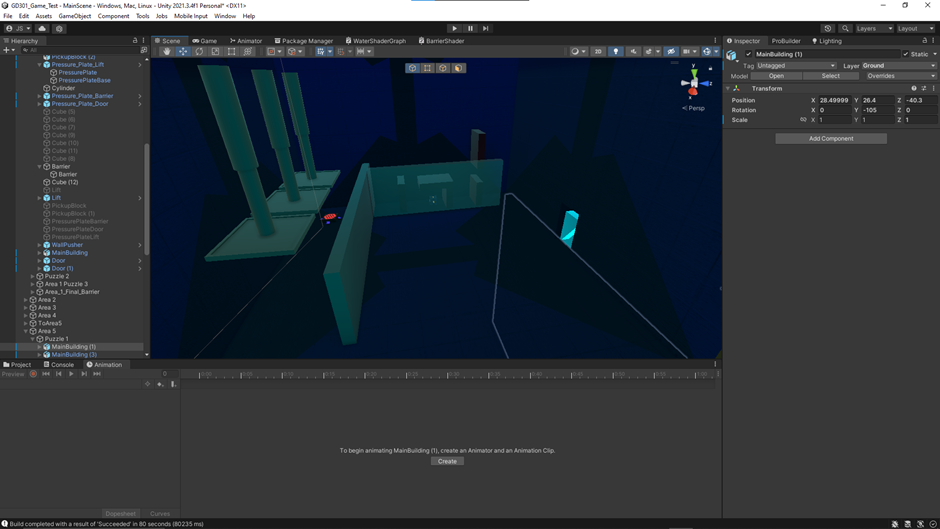
This is the new pickup script that I have entirely rewritten where it detects the pick-up layer and moves it into a transform in front of the player.
public class PickUpScriptFinal : MonoBehaviour
{
[SerializeField] private Transform pickUpTransform;
[SerializeField] private Camera playerCamera;
[SerializeField] private LayerMask pickUpMask;
[Space]
[SerializeField] public float pickupRange = 4f;
public bool isHolding;
//public RaycastHit hit;
public Rigidbody currentObject;
private Vector3 turn;
private void Update()
{
if (Input.GetKeyDown(KeyCode.E))
{
if (currentObject)
{
currentObject.freezeRotation = false;
currentObject.useGravity = true;
currentObject = null;
isHolding = false;
Debug.Log("Picked Up");
return;
//this to drop object
}
Ray CameraRay = playerCamera.ViewportPointToRay(new Vector3(0.5f, 0.5f, 0f));
if (Physics.Raycast(CameraRay, out RaycastHit HitInfo, pickupRange, pickUpMask))
{
currentObject = HitInfo.rigidbody;
currentObject.useGravity = false;
isHolding = true;
currentObject.freezeRotation = true;
//GameObject.Find("FirstPersonCharacterCamera").GetComponent<MouseMovement>().enabled = true;
//This part to pick object up
}
}
if (isHolding)
{
if (Input.GetKey(KeyCode.R))
{
Debug.Log("Block Rotate");
turn.x += Input.GetAxis("Mouse X");
turn.y += Input.GetAxis("Mouse Y");
//GameObject.Find("FirstPersonCharacterCamera").GetComponent<MouseMovement>().enabled = false;
currentObject.transform.localRotation = Quaternion.Euler(-turn.y * 5, turn.x * 5, 0);
}
else
{
//GameObject.Find("FirstPersonCharacterCamera").GetComponent<MouseMovement>().enabled = false;
}
if (Input.GetKeyUp(KeyCode.R))
{
currentObject.freezeRotation = true;
currentObject.freezeRotation = false;
}
}
}
public void FixedUpdate()
{
if (isHolding)
{
Vector3 DirectionToPoint = pickUpTransform.position - currentObject.position;
float DistanceToPoint = DirectionToPoint.magnitude;
currentObject.velocity = DirectionToPoint * 12f * DistanceToPoint;
}
else
{
return;
}
}
This is the script for the Magnet Power where if you hold both mouse buttons down it will turn into a magnet which can pull boxes towards the player or it also allows the player to magnetise to walls.
public Transform magnetTransform;
public PickUpScriptFinal _currectObject;
public PlayerElectricity playerElectricity;
public DoubleElectricity doubleElectricity;
public ParticleSystem electricityPulse;
public ParticleSystem doubleElectricityPulse;
private Rigidbody _currentObject;
[SerializeField] private Camera playerCamera;
[SerializeField] public float pickupRange = 4f;
[SerializeField] private LayerMask pickUpMask;
private bool isHolding;
public SphereCollider sphereCollider;
private void Start()
{
playerElectricity.GetComponent<PlayerElectricity>();
doubleElectricity.GetComponent<DoubleElectricity>();
electricityPulse.GetComponent<ParticleSystem>();
doubleElectricityPulse.GetComponent<ParticleSystem>();
sphereCollider.GetComponent<SphereCollider>();
}
private void Update()
{
if (playerElectricity.isOn && doubleElectricity.isOn == true && Input.GetKey(KeyCode.Mouse0) && Input.GetKey(KeyCode.Mouse1))
{
var col = electricityPulse.collision;
col.enabled = false;
var col2 = doubleElectricityPulse.collision;
col2.enabled = false;
sphereCollider.enabled = true;
Ray CameraRay = playerCamera.ViewportPointToRay(new Vector3(0.5f, 0.5f, 0f));
if (Physics.Raycast(CameraRay, out RaycastHit HitInfo, pickupRange, pickUpMask))
{
Debug.Log("PickUpMagnet");
_currentObject = HitInfo.rigidbody;
_currentObject.useGravity = false;
_currentObject.freezeRotation = true;
isHolding = true;
}
}
else if(_currentObject)//(Input.GetKeyUp(KeyCode.Mouse0) && Input.GetKeyUp(KeyCode.Mouse1) && playerElectricity.isOn && doubleElectricity.isOn == false)
{
var col = electricityPulse.collision;
col.enabled = true;
var col2 = doubleElectricityPulse.collision;
col2.enabled = true;
sphereCollider.enabled = false;
//-------Drop-------
Debug.Log("DroppedMagnet");
_currentObject.freezeRotation = false;
_currentObject.useGravity = true;
isHolding = false;
_currentObject = null;
return;
}
else
{
var col = electricityPulse.collision;
col.enabled = true;
var col2 = doubleElectricityPulse.collision;
col2.enabled = true;
}
}
void FixedUpdate()
{
if (isHolding)
{
Vector3 DirectionToPoint = magnetTransform.position - _currentObject.position;
float DistanceToPoint = DirectionToPoint.magnitude;
_currentObject.velocity = DirectionToPoint * 12f * DistanceToPoint;
}
}
private void OnTriggerStay(Collider other)
{
if (other.gameObject.tag == "MagneticWall" && playerElectricity.isOn && doubleElectricity.isOn == true && Input.GetKey(KeyCode.Mouse0) && Input.GetKey(KeyCode.Mouse1))
{
transform.position = Vector3.MoveTowards(transform.position, other.ClosestPoint(Vector3.forward), 15 * Time.deltaTime);
magnetTransform.GetComponent<SphereCollider>().enabled = true;
}
else if (playerElectricity.isOn && doubleElectricity.isOn == false && Input.GetKeyUp(KeyCode.Mouse0) && Input.GetKeyUp(KeyCode.Mouse1))
{
magnetTransform.GetComponent<SphereCollider>().enabled = false;
return;
}
}
private void OnTriggerExit(Collider other)
{
if (other.gameObject.tag == "Box")
{
Debug.Log("Drop");
//other.transform.position = magnet.transform.position;
//_isHolding.hit.collider.transform.parent = null;
}
}