This is a simple main menu screen I created so far.
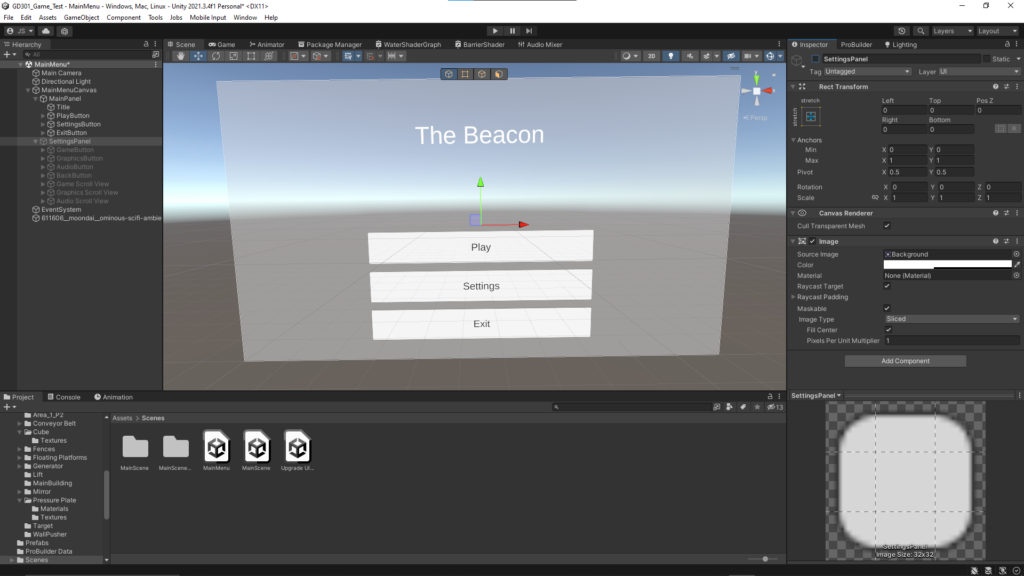
This is the settings menu that I’ve made so far. It has 3 areas that you can select, ‘Game’, ‘Graphics’ and ‘Audio’. In ‘Game’, there is a slider for the FOV and one for the Sensitivity. I’ve done this so players can customise these settings to how they like. In ‘Graphics’, there is a dropdown menu for resolution, a fullscreen toggle and a Vsync toggle. In ‘Audio’, there is a slider for Music and a slider for SFX. I might make one for Master volume but for now these will do.
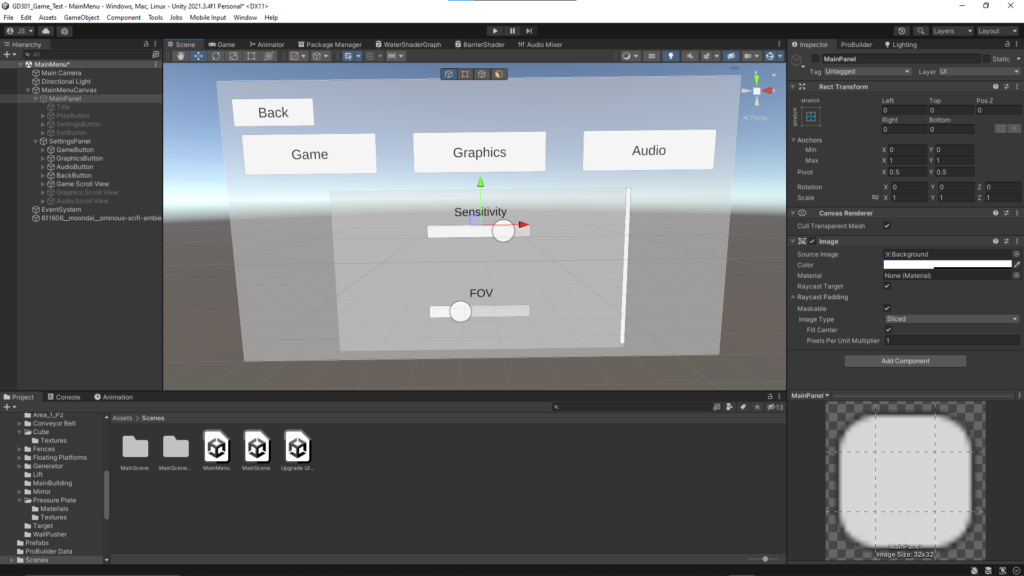
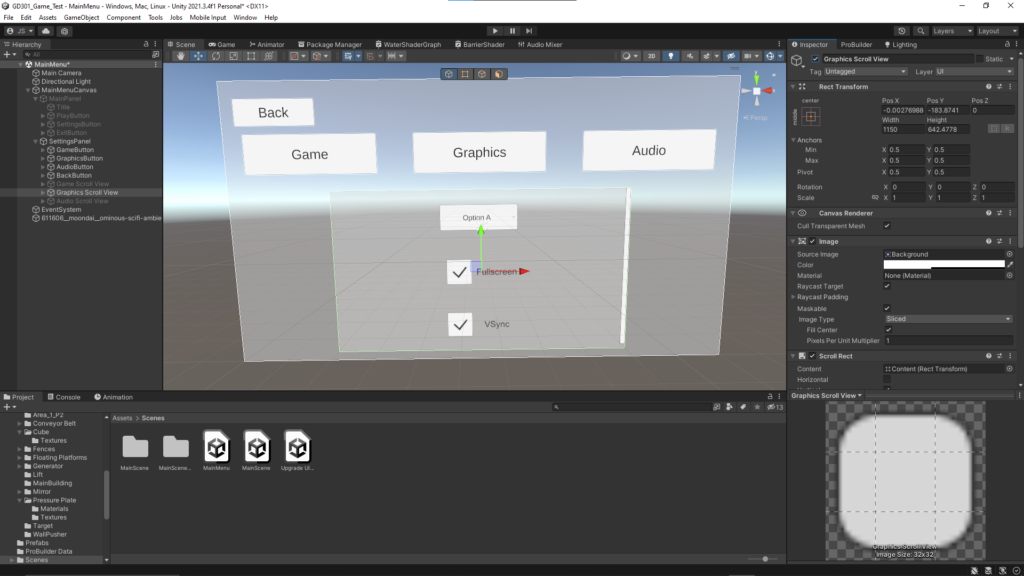
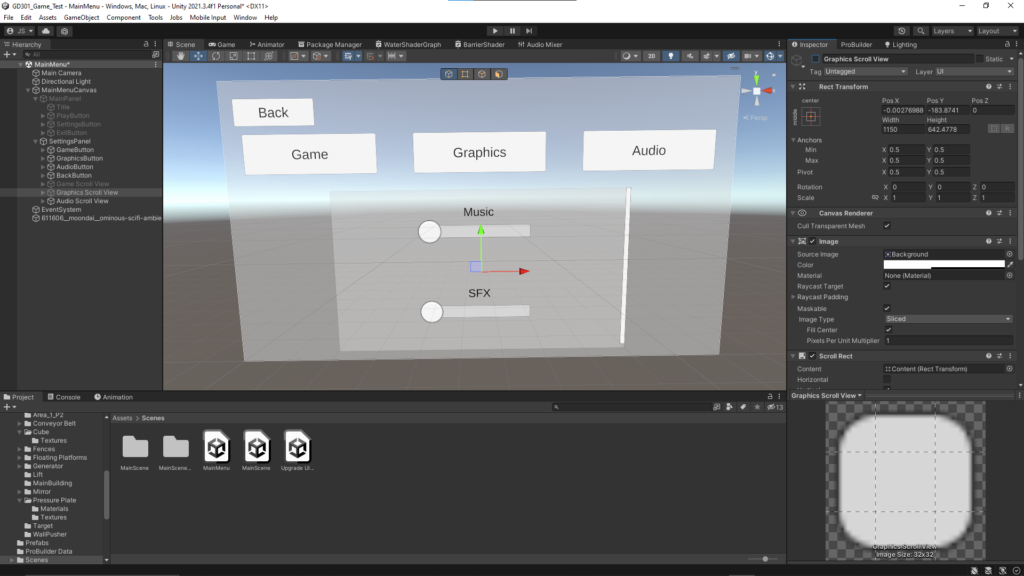
This is the script for the main menu so far. I’ve tried to make it as neat and tidy as possible so it is easy to see where each section is.
public class MainMenuScript : MonoBehaviour
{
[Header("UI")]
public GameObject[] panels;
public GameObject graphicsScrollView;
public GameObject audioScrollView;
public GameObject gameScrollView;
[Header("Resolution")]
public TMP_Dropdown resolutionDropdown;
Resolution[] resolutions;
[Header("Audio")]
public AudioMixer masterMixer;
public Slider musicSlider, sfxSlider;
[Header("Sensitivity")]
public float sensitivityValue;
public Slider fovSlider;
public float fovValue;
private void Start()
{
resolutions = Screen.resolutions;
resolutionDropdown.ClearOptions();
List<string> options = new List<string>();
int currentResolutionIndex = 0;
for (int i = 0; i < resolutions.Length; i++)
{
string option = resolutions[i].width + " x " + resolutions[i].height;
options.Add(option);
if (resolutions[i].width == Screen.currentResolution.width &&
resolutions[i].height == Screen.currentResolution.height)
{
currentResolutionIndex = i;
}
}
resolutionDropdown.AddOptions(options);
resolutionDropdown.value = currentResolutionIndex;
resolutionDropdown.RefreshShownValue();
}
public void Play()
{
SceneManager.LoadScene("MainScene");
}
public void SettingsPanel()
{
panels[0].SetActive(false);
panels[1].SetActive(true);
}
public void GamePanel()
{
audioScrollView.SetActive(false);
graphicsScrollView.SetActive(false);
gameScrollView.SetActive(true);
}
//------------Game-------------------
public void Sensitivity(float sensitivity)
{
sensitivityValue = sensitivity;
PlayerPrefs.SetFloat("currentSensitvity", sensitivity);
}
public void SensitivitySlider()
{
PlayerPrefs.SetFloat("SensitivitySlider", sensitivityValue);
Debug.Log(sensitivityValue);
}
public void FOVSlider()
{
Camera.main.fieldOfView = fovSlider.value;
}
public void FOV(float fov)
{
fovValue = fov;
PlayerPrefs.SetFloat("FOV", fov);
}
//------------Game-------------------
//-----------Graphics----------------
public void GraphicsPanel()
{
audioScrollView.SetActive(false);
graphicsScrollView.SetActive(true);
gameScrollView.SetActive(false);
}
public void SetResolution(int resolutionIndex)
{
Resolution resolution = resolutions[resolutionIndex];
Screen.SetResolution(resolution.width, resolution.height, Screen.fullScreen);
}
public void FullscreenToggle()
{
Screen.fullScreen = !Screen.fullScreen;
}
public void VSync(bool boxChecked)
{
if (boxChecked)
{
QualitySettings.vSyncCount = 1;
}
else
{
QualitySettings.vSyncCount = 0;
}
}
//-----------Graphics----------------
//------------Audio----------------
public void AudioPanel()
{
audioScrollView.SetActive(true);
graphicsScrollView.SetActive(false);
gameScrollView.SetActive(false);
}
public void MusicSlide()
{
PlayerPrefs.SetFloat("MusicSlider", musicSlider.value);
}
public void SFXSlide()
{
PlayerPrefs.SetFloat("MusicSlider", sfxSlider.value);
}
public void MusicVolume(float volume)
{
masterMixer.SetFloat("MusicVol", Mathf.Log10(volume) * 20);
}
public void SFXVolume(float volume)
{
masterMixer.SetFloat("SFXVol", Mathf.Log10(volume) * 20);
}
//------------Audio----------------
public void Back()
{
panels[0].SetActive(true);
panels[1].SetActive(false);
graphicsScrollView.SetActive(false);
}
public void Exit()
{
Application.Quit();
Debug.Log("Quit");
}
Conveyor Belt
For the arrows, I added emission onto them to give them a more sci-fi look to them.
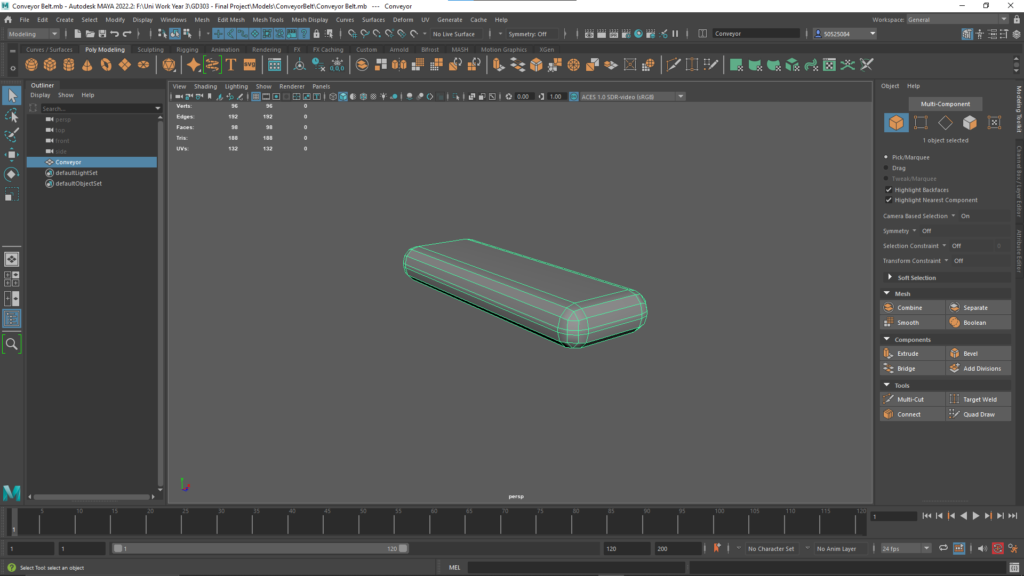
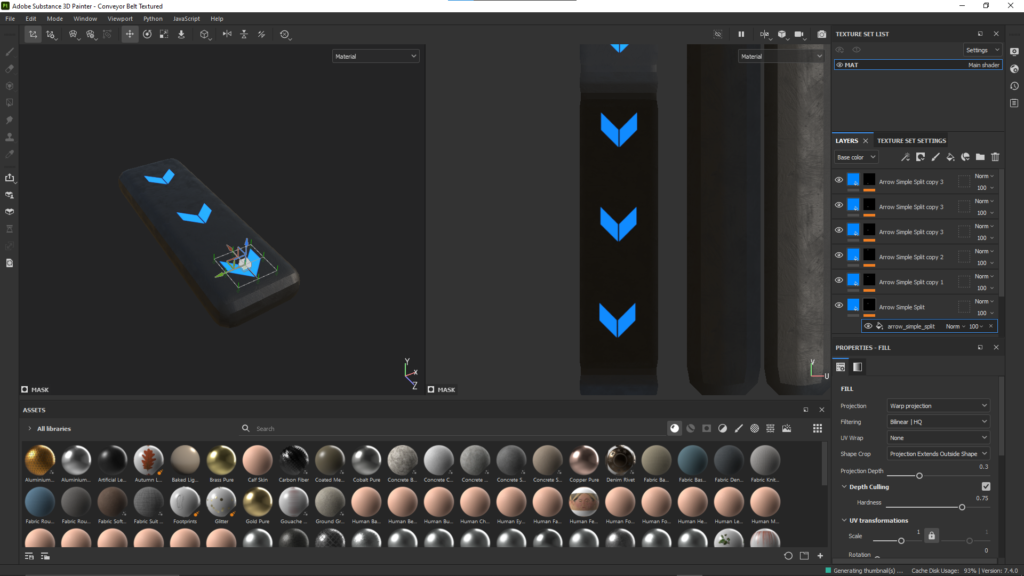
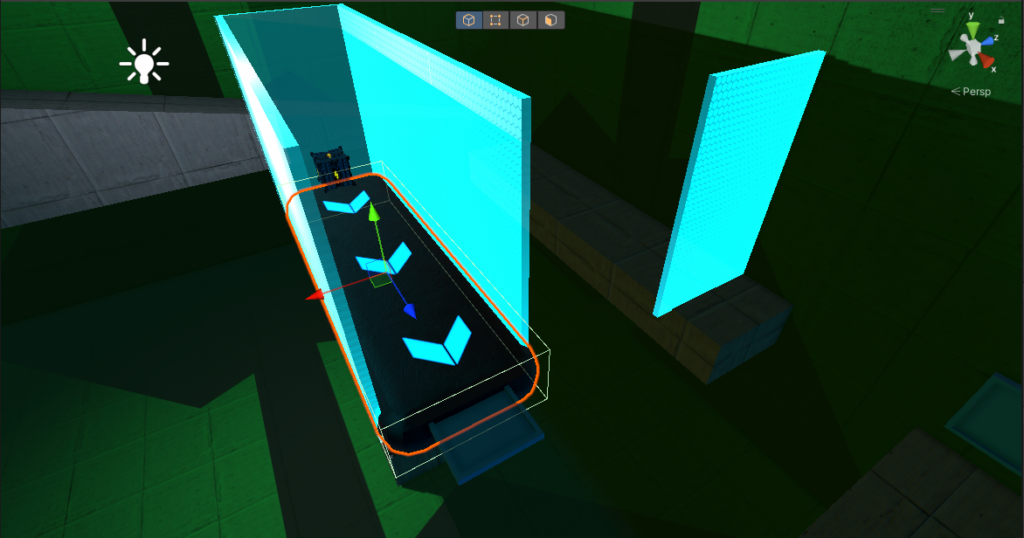
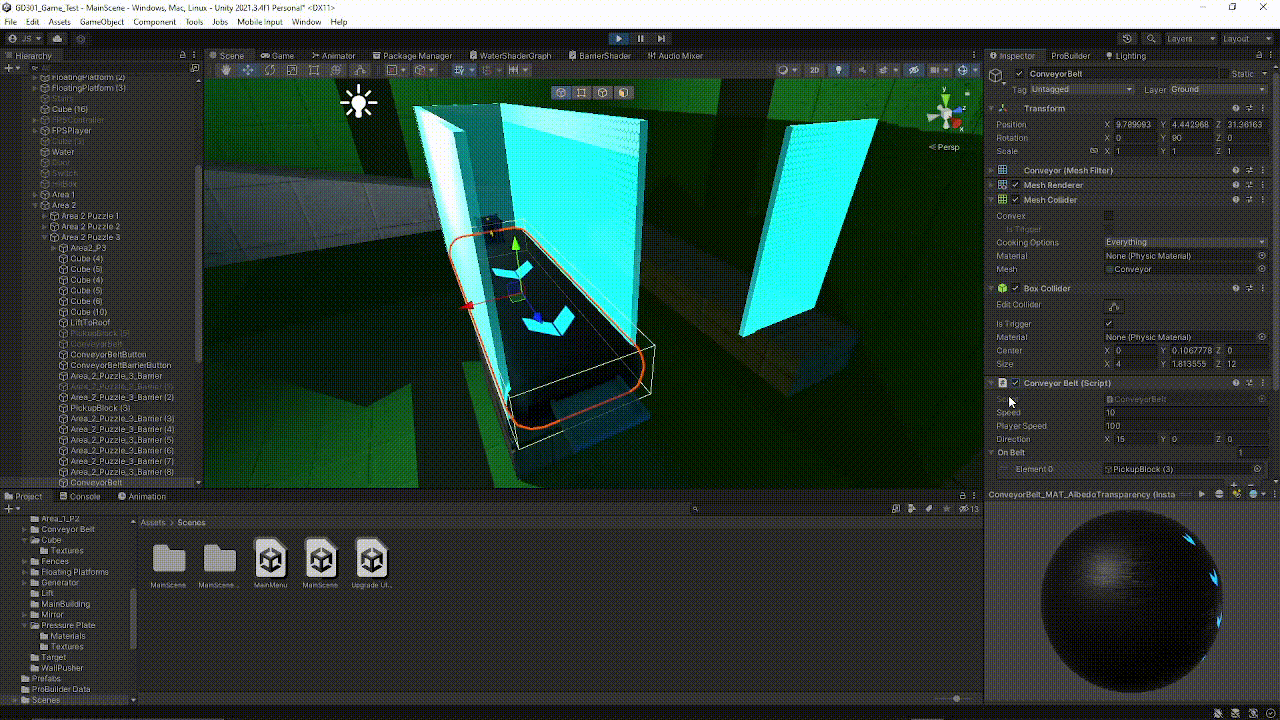
I made the texture scroll to make it look like it moves.
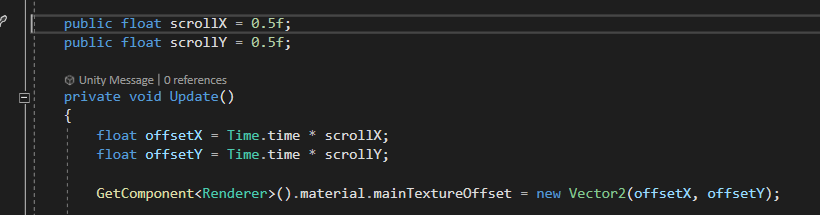
There was an issue with the collision on the conveyor belt with the player where it would not register the player being on it. I fixed this by changing the code from OnCollision to OnTrigger and made a trigger box bigger on the conveyor belt and now it works perfectly.
I made a reticle which fills in once you pick up an object. I did this to make it so the player knows that they have definitely picked up a cube.
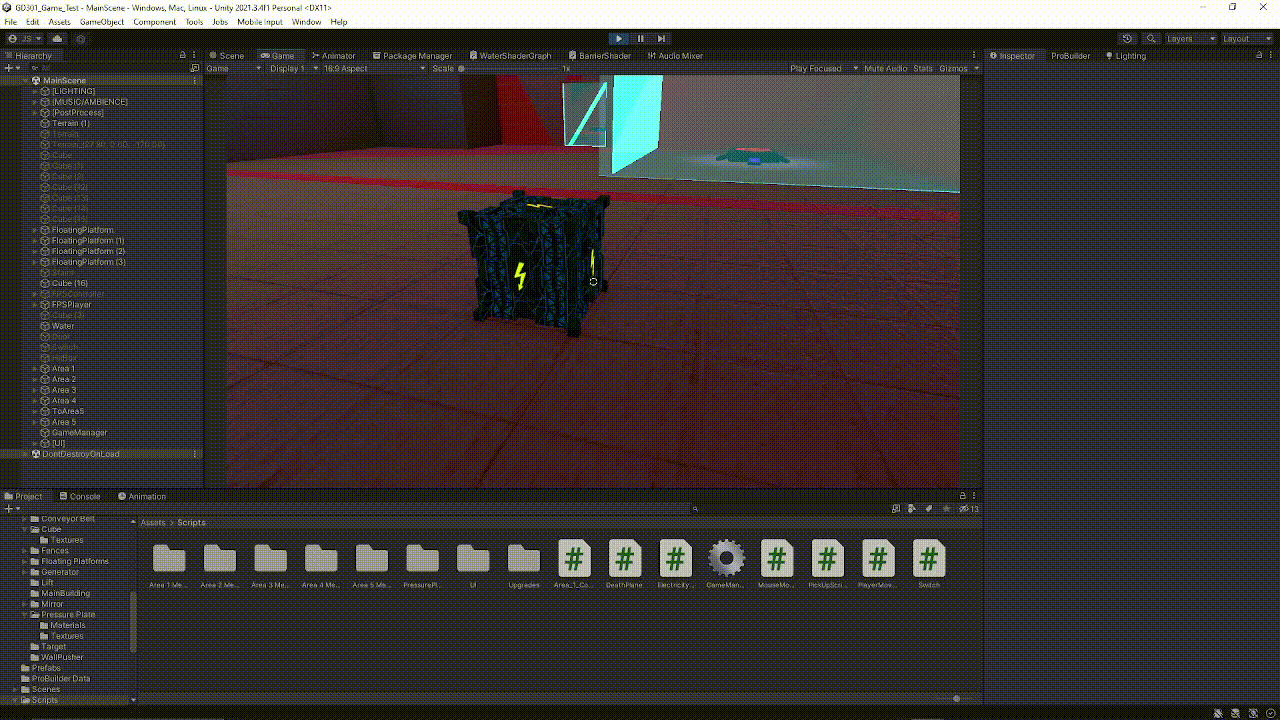
This is the starting message that will be displayed at the start which was something someone suggested in my survey as they didn’t know what the controls or story were so this is to help players know a bit more about why the player is here and how to control the player.
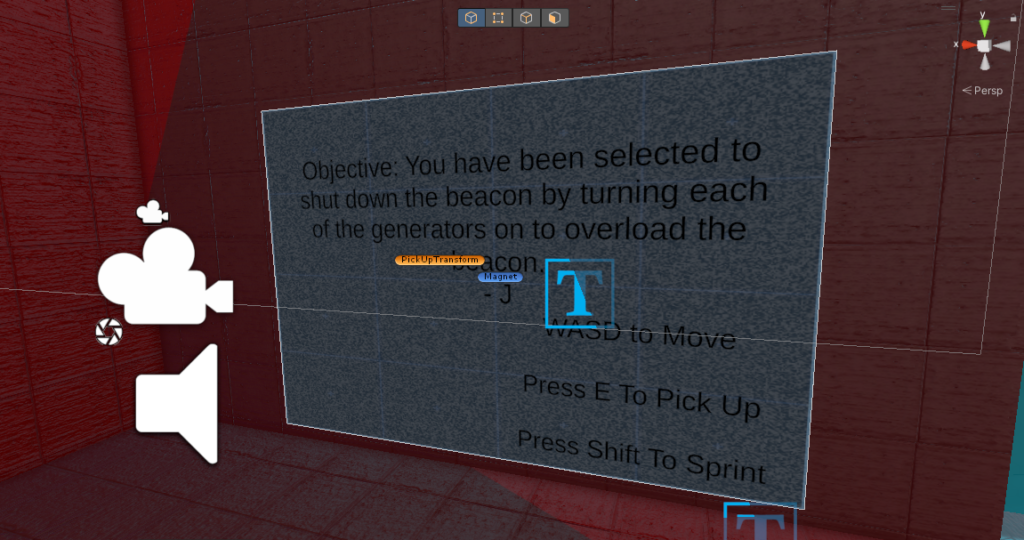
This is the script for the screen which loops the texture and also does the typewriter text.
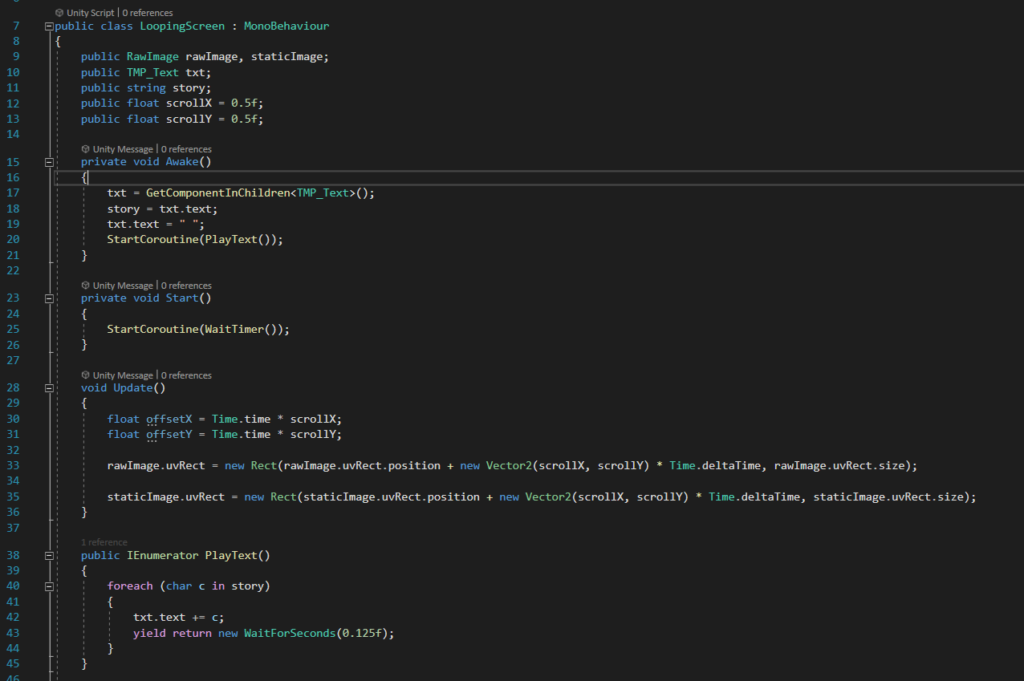
Here is a GIF of this in action.
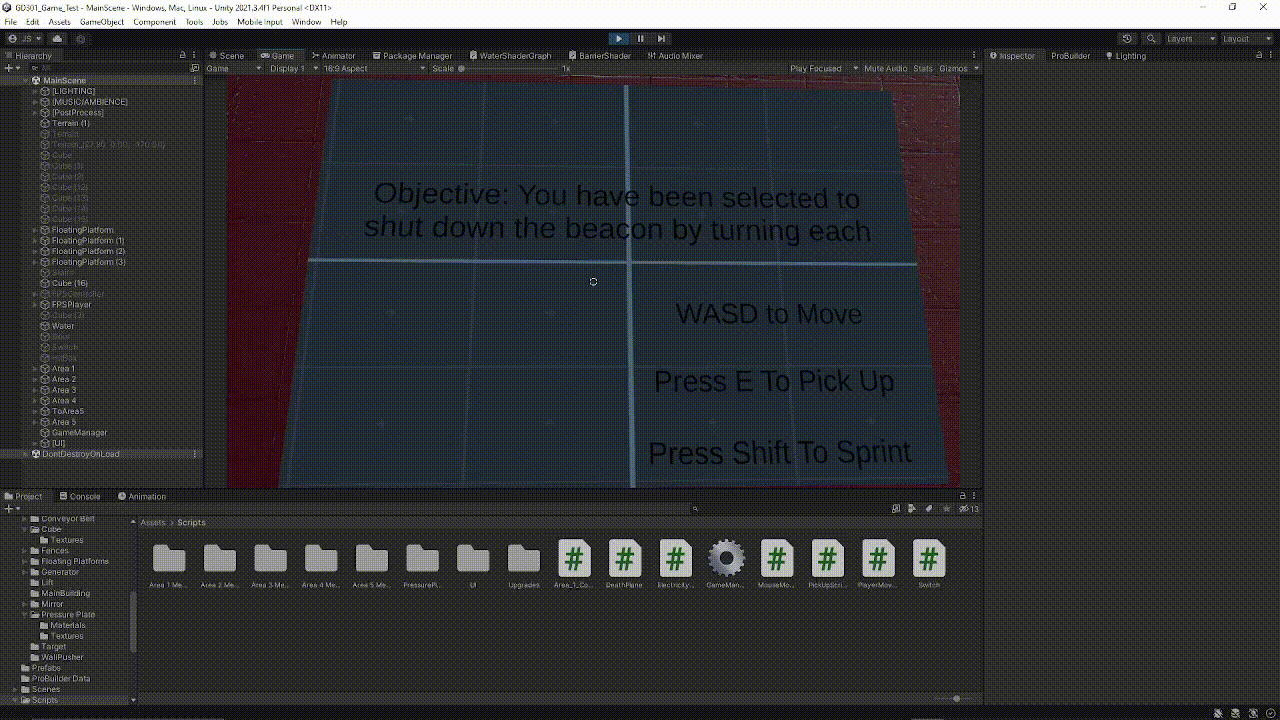
Sound Effects
Sci Fi Button Press – https://freesound.org/people/peepholecircus/sounds/196979/
Correct Beep – https://freesound.org/people/Bertrof/sounds/131660/
Door Open/Close – https://freesound.org/people/primeval_polypod/sounds/156509/